HTML Forms
HTML forms are used to collect data from user.
Form in HTML is defined with the <form> element.
The form contains other elements such as text boxes, radio buttons, check boxes etc.
The form itself is not visible, only the elements are visible.
HTML Basic Form(Difficulty Level - Beginner)
Write or copy some HTML into your editor.
<html>
<body>
<form>
Full Name: <br>
<input type= "text" name= "fullname" value= "Enter your full name" > <br>
Email: <br>
<input type= "email" name= "email" value= "Enter your email address" > <br>
<input type= "submit" name= "subscribe" value= "Subscribe" >
</form>
</body>
</html>
View the HTML Page in Your Browser
Open the saved HTML file in your favorite browser (double click on the file, or right-click - and choose "Open with").
The result will be similar to following figure.
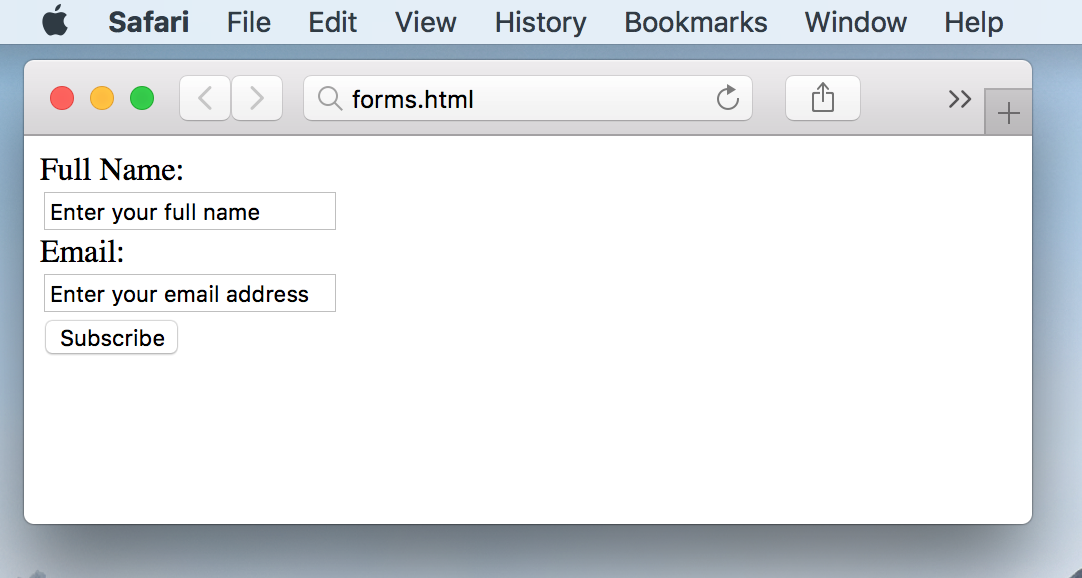
Various Input elements of HTML Forms
HTML Form Inputs ...
Text
Passwords
Radio Buttons
Check Boxes
The Text Element of HTML Form
The text element defines a single line input field for text
It is defined by <input type="text">
The default width of a text field is 20 characters.
Write or copy some HTML into your editor.
<html>
<body>
<form>
First Name: <br>
<input type= "text" name= "firstname" value= "Enter your first name" > <br>
Last Name: <br>
<input type= "text" name= "lasttname" value= "Enter your last name" > <br>
<input type= "submit" name= "subscribe" value= "Subscribe" >
</form>
</body>
</html>
View the HTML Page in Your Browser
Open the saved HTML file in your favorite browser (double click on the file, or right-click - and choose "Open with").
The result will be similar to following figure.
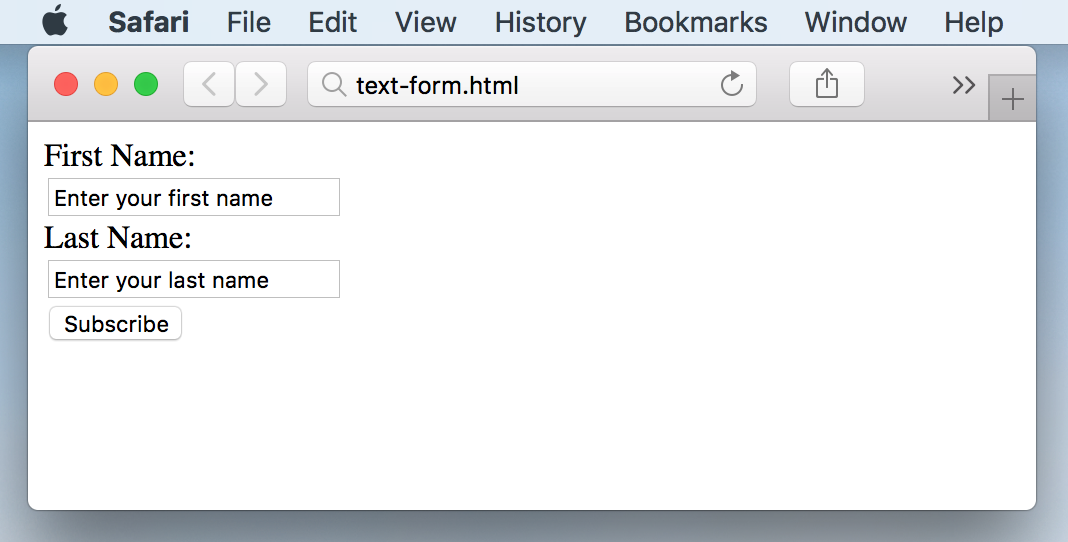
The Radio Element of HTML Form
Radio buttons allows us to select ONE of many choices:
It is defined by <input type="radio">
Write or copy some HTML into your editor.
<html>
<body>
<form>
<input type= "radio" name= "actor" value= "Harry Potter" > Harry Potter <br>
<input type= "radio" name= "actor" value= "Hermione Granger" > Hermione Granger <br>
<input type= "radio" name= "actor" value= "Ronald Weasley" > Ronald Weasley
</form>
</body>
</html>
View the HTML Page in Your Browser
Open the saved HTML file in your favorite browser (double click on the file, or right-click - and choose "Open with").
The result will be similar to following figure.
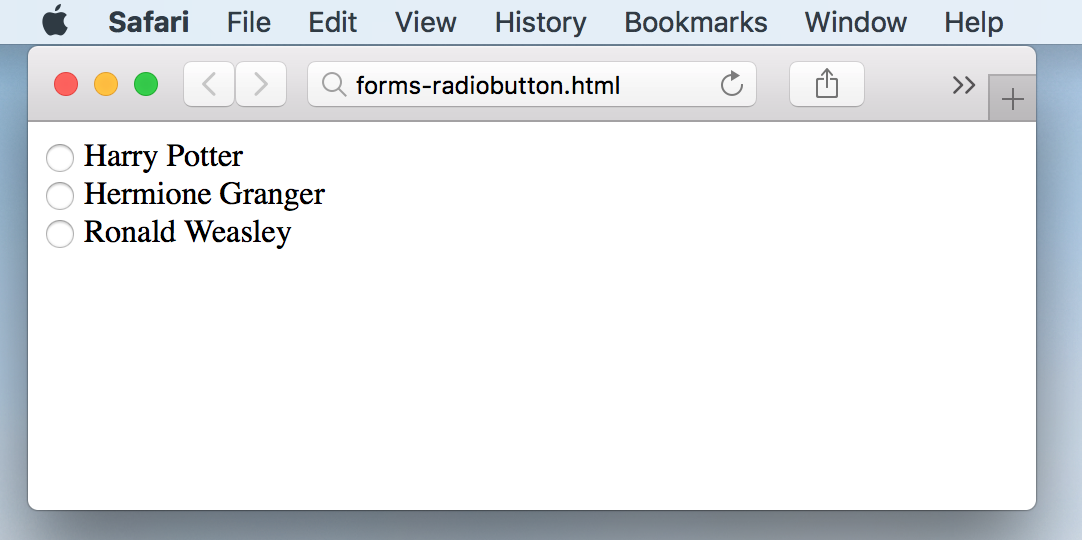
Form Submission
The forms are generally submitted to back-end servers.
Submit Button submits the form to form handler
Action Attribute defines the form handler
Method Attribute defines the POST or GET Method
Target Attribute defines wether the result will be opened in new or same page
The Action Attribute of HTML Form
The action on forms is generally taken at the back-end servers.
It is defined by <form action="/form-handler.php">
Form handler is generally a script and is defined in the form's action attribute.
The Method Attribute of HTML Form
The Method Attribute specifies which HTTP method (GET or POST) to be used for submission of form.
By default the value of method attribute is "GET"
GET method is defined by <form action="/form-handler.php" method="get">
POST method is defined by <form action="/form-handler.php" method="post">
GET
The submitted data is shown in the URL.
The data which can be passed with "GET" is Limited (generally less than 3000 characters)
The results from "GET" can be Bookmarked
POST
In "POST" method, the data is hidden
The data passed with "POST", generally has no limits
The results from "POST" can NOT be Bookmarked
The Submit Element of HTML Form
Submit button, submits the form to the form handler (back-end server).
It is defined by <input type="submit">
Write or copy some HTML into your editor.
<html>
<body>
<form action="/register.php">
Full Name: <br>
<input type= "text" name= "fullname" value= "Enter your full name" > <br>
Email: <br>
<input type= "email" name= "email" value= "Enter your email address" > <br>
<input type= "submit" name= "subscribe" value= "Subscribe" >
</form>
</body>
</html>
View the HTML Page in Your Browser
Open the saved HTML file in your favorite browser (double click on the file, or right-click - and choose "Open with").
The result will be similar to following figure.
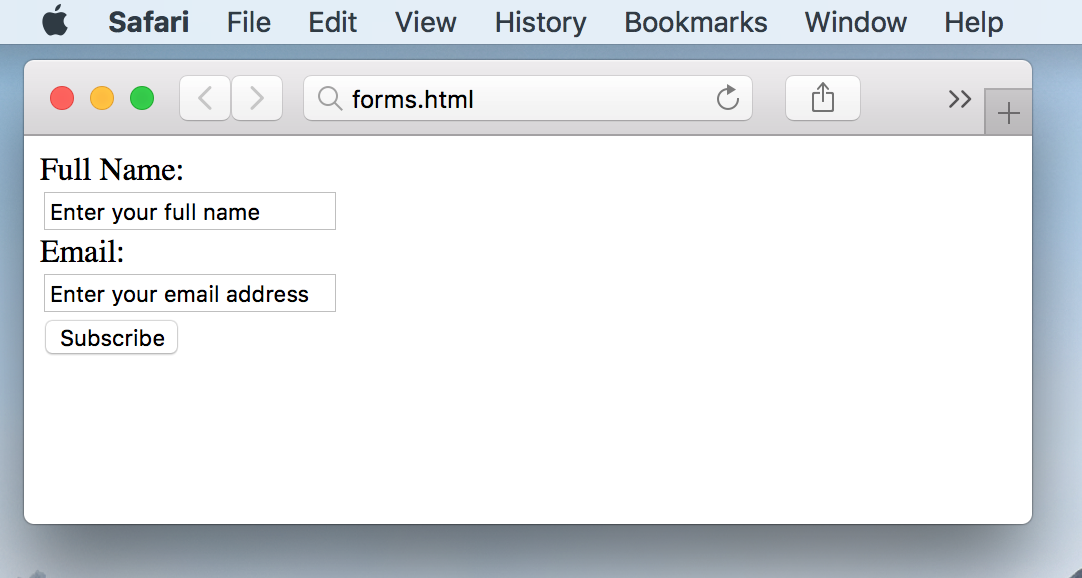
The Target Attribute of HTML Form
The Target Attribute tells the browser to open the result of submitted form in the current window, new tab or a new frame.
By default the value of target attribute is "_self" and the result of submitted form will be available in current window
It is defined by <form action="/form-handler.php" target="_blank">
target="_self" opens result of form in same window. (Default Settings)
target="_blank" opens result of form in new window.
target="_parent" opens result of form in parent window.
target="_top" opens result of form in top window.
target="Name of Iframe" opens result of form in an iframe.